Long before anyone considered standardizing how browsers would handle events, Netscape Communications Corporation introduced an event-handling model in its Netscape Navigator browser. This model is known by a few names. You may have heard it termed the Netscape Event Model, the Basic Event Model, or even the rather vague Browser Event Model, but most people have come to call it the DOM Level 0 Event Model.
Note
The term DOM level is used to indicate what level of requirements an implementation of the W3C DOM specification meets. There isn’t a DOM Level 0, but that term is used to informally describe what was implemented prior to DOM Level 1.
The W3C didn’t create a standardized model for event handling until DOM Level 2, introduced in November 2000. This model enjoys support from all modern standards-compliant browsers such as Internet Explorer 9 and above, Firefox, Chrome, Safari, and Opera. Internet Explorer 8 and below have their own way and support a subset of the functionality in the DOM Level 2 Event Model, albeit using a proprietary interface.
Before we show you how jQuery makes that irritating fact a non-issue, you need to spend some time getting to know how the various event models operate.
The DOM Level 0 Event Model
The DOM Level 0 Event Model is the event model that most amateurs and beginning web developers employ on their pages because it’s somewhat browser-independent and fairly easy to use.
Under this event model, event handlers are declared by assigning a reference to a function instance to properties of the DOM elements. These properties are defined to handle a specific event type; for example, a click event is handled by assigning a function to the onclick property and a mouseover event by assigning a function to the onmouseover property of elements that support these event types.
The browsers also allow you to specify the body of event handler functions as attribute values embedded within the HTML markup of the DOM elements, providing a shortcut for creating event handlers. An example of defining handlers in both ways is shown in the following listing, which can be found in the downloadable code for this app in the file lesson-6/dom.0.events.html.
Listing 6.1. Declaring DOM Level 0 event handlers
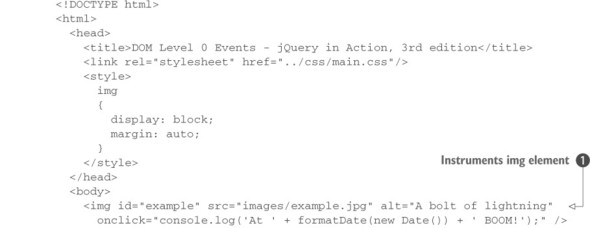
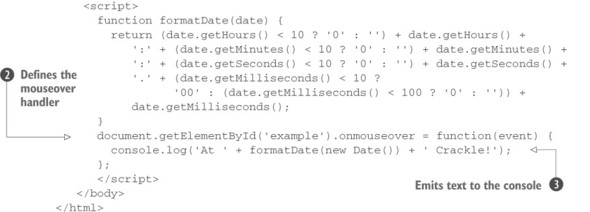
In this example, you employ both styles of event handler declaration: declaring in a markup attribute and declaring under script control
. In the body of the page, you define an img element, having example as its ID, upon which you’re defining the event handler for the click event using the onclick attribute
.
Inside the <script> tag, you declare a function called formatDate() used to format and return a string representing the time of the given Date object. Then, using the JavaScript’s getElementById() method, you retrieve a reference to the image, setting its onmouseover property to an inline function . This function becomes the event handler for the element when a mouseover event is triggered on it. Note that this function expects a single parameter to be passed to it, which we’ll discuss in a few moments. Within this function, you print on the console a formatted date using once again the previously declared formatDate() function
.
Note
We’ve thrown the concept of unobtrusive JavaScript out the window for this example. Long before you reach the end of this lesson, you’ll see why you won’t need to embed event behavior in the DOM markup anymore!
Loading this page (found in the file lesson-6/dom.0.events.html) into Chrome, waving the mouse pointer over the image a few times, and then clicking the image results in a display similar to that shown in figure 6.1.
Figure 6.1. Waving the mouse over the image and clicking it results in the event handlers firing and emitting their messages to the console.
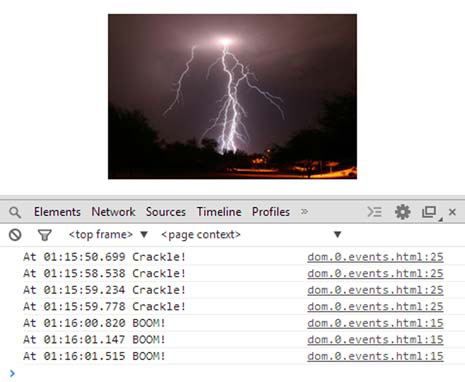
You declared the click event handler in the img element markup using the following attribute:
onclick="console.log('At ' + formatDate(new Date()) + ' BOOM!');"
This might lead you to believe that the statement becomes the click event handler for the element, but that’s not the case. When handlers are declared via HTML markup attributes, an anonymous function is automatically created using the value of the attribute as the function body. Assuming that imageElement is a reference to the image element, the construct created as a result of the attribute declaration is equivalent to the following:
imageElement.onclick = function(event) {
console.log('At ' + formatDate(new Date()) + ' BOOM!');
};
Note how the value of the attribute is used as the body of the generated function and that the function is created so that the event parameter is available within the generated function. Finally, within this function you can refer to the element itself using the this keyword. We haven’t used it in our simple example, but it’s a detail we wanted to highlight.
Once again, we want to remind you that using the attribute mechanism of declaring DOM Level 0 event handlers violates the precepts of unobtrusive JavaScript. You’ll shortly see that JavaScript and jQuery, which also deal with browser incompatibilities, provide a much better way to declare event handlers than either of the means described. But first, let’s examine what that event parameter is all about.
Leave a Reply