You saw earlier that with the DOM Level 0 Event Model, once an event was triggered on an element, the event propagated from the target element upward in the DOM tree to all the target’s ancestors. The advanced Level 2 Event Model also provides this bubbling phase but ups the ante with an additional capture phase.
Under the DOM Level 2 Event Model, when an event is triggered, the event first propagates from the root of the DOM tree down to the target element and then propagates again from the target element up to the DOM root. The former phase (root to target) is called the capture phase, and the latter (target to root) is called the bubble phase.
When a function is established as an event handler, it can be flagged as a capture handler, in which case it will be triggered during the capture phase, or as a bubble handler, to be triggered during the bubble phase. As you might have guessed by this time, the useCapture parameter to addEventListener() identifies which type of handler is established. A value of false for this parameter establishes a bubble handler, whereas a value of true registers a capture handler. This parameter has become optional, defaulting to false in newer versions of all major browsers (for example, in Firefox starting from version 6).
Think back a moment to the example of listing 6.2 where you explored the propagation of the Basic Model events through a DOM hierarchy. In that example, you embedded an image element within three layers of div elements. Within such a hierarchy, under DOM Level 2, the propagation of a click event with the img element as its target would move through the DOM tree as shown in figure 6.4.
Figure 6.4. Propagation in the DOM Level 2 Event Model traverses the DOM hierarchy twice: once from top to target during the capture phase and once from target to top during the bubble phase.
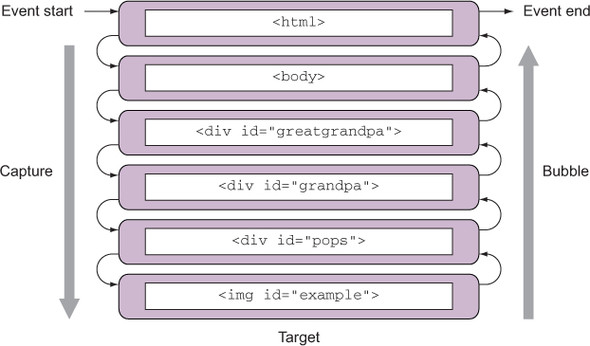
Let’s put that to the test, shall we? The next listing shows the code for a page containing the element hierarchy of figure 6.4 (lesson-6/dom.2.propagation.html).
Listing 6.4. Tracking event propagation with bubble and capture handlers
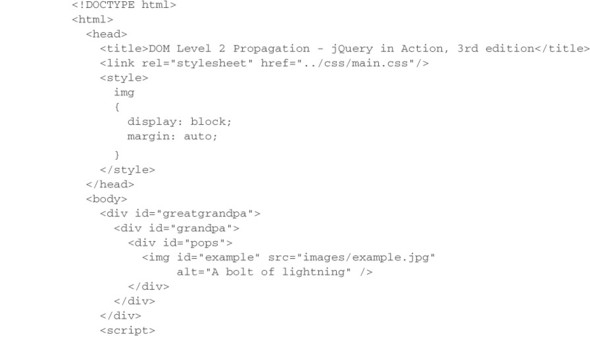
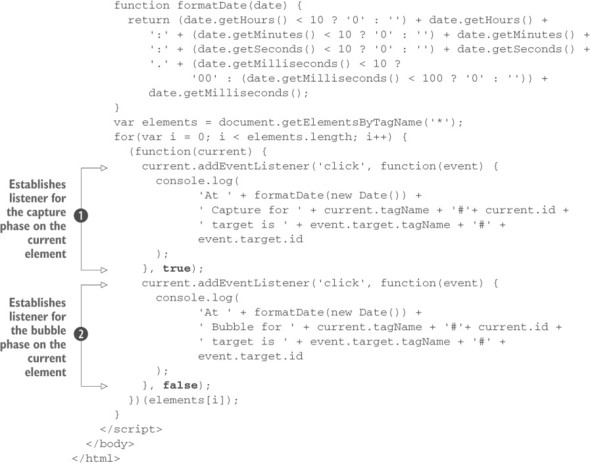
This code changes the example of listing 6.2 to use the DOM Level 2 Event Model to establish the event handlers. In the <script> tag, you use the getElementsByTagName() method and the Universal selector to retrieve all the elements on the page. On each of them, you establish two handlers: one capture handler and one bubble handler
. Each handler prints a message on the console identifying the type of handler, the current element, and the ID of the target element.
With the page loaded into Chrome, clicking the image results in the display in figure 6.5 showing the progression of the event through the handling phases and the DOM tree. Note that because you defined both capture and bubble handlers for the target, two handlers were executed for the target and all its ancestor nodes.
Figure 6.5. Clicking the image results in each handler emitting a console message that identifies the path of the event during both the capture and bubble phases.
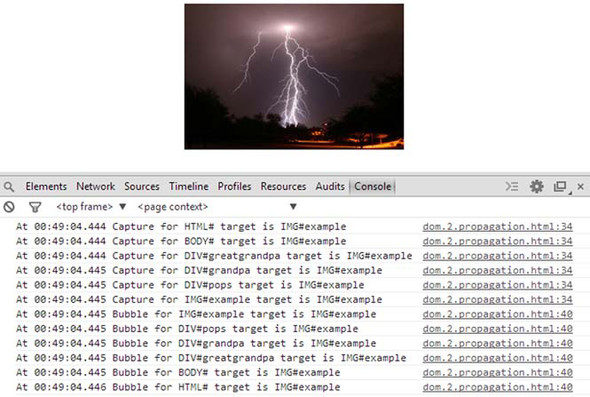
Now that we’ve put you through all the trouble to understand these two types of handlers, you should know that capture handlers are hardly ever used in web pages. One of the historical reasons is that old versions of Internet Explorer don’t support this type of event propagation.
Before we look at how jQuery can help sort out this mess, let’s briefly examine the Internet Explorer Model.
The Internet Explorer Model
Versions of Internet Explorer prior to 9 don’t provide support for the DOM Level 2 Event Model. These versions of Microsoft’s browser provide a proprietary interface that closely resembles the bubble phase of the standard model. Rather than add-EventListener(), the Internet Explorer Model defines a method named attach-Event() for each DOM element. This method accepts two parameters similar to those of the standard model:
attachEvent(eventName, handler)
The first parameter is a string that names the event type to be attached but uses the name of the corresponding element property from the DOM Level 0 Model such as onclick, onmouseover, onkeydown, and so on.
The second parameter is the function to be established as the handler, and the Event instance must be fetched from the window.event property. In addition, this method doesn’t support any semblance of a capture phase.
Even when using the relatively browser-independent DOM Level 0 Model, you’re faced with a tangle of browser-dependent choices to make at each stage of event handling. And when using the more capable DOM Level 2 or Internet Explorer Model, you even have to fork your code when establishing the handlers in the first place if you want to support a wider audience.
jQuery is going to simplify your life by hiding these browser disparities for you. Let’s see how!
Leave a Reply