Sometimes you need to perform a given action or repeat it over and over again after a given amount of time. Other times you want to retrieve information from a server without reloading the page. These are situations where you need to execute one or more functions asynchronously.
To test asynchronous functions you can use the same method to create the test and the same assertion methods you learned in the previous section. But you also need a mechanism to inform the test runner that you’re waiting for an asynchronous method to be completed. Let’s look at the async() method. It belongs to the same assert parameter we’ve mentioned many times in this lesson, and its syntax is the following.
Method syntax: async | |
---|---|
async() | |
Instruct QUnit to wait for an asynchronous operation. | |
Parameters | |
none | |
Returns | |
A unique resolution callback function. |
As you can see from the description, this method doesn’t accept any parameters and returns a function. This function is unique and must be used only once, and it must be invoked inside the asynchronous function you want to test.
To better understand how this methods works, let’s analyze the code shown in the next listing, which is also available in the file lesson-14/asynchronous.test.html.
Listing 14.3. Asynchronous test with QUnit
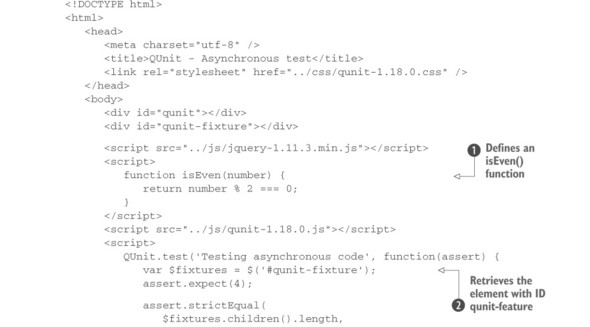
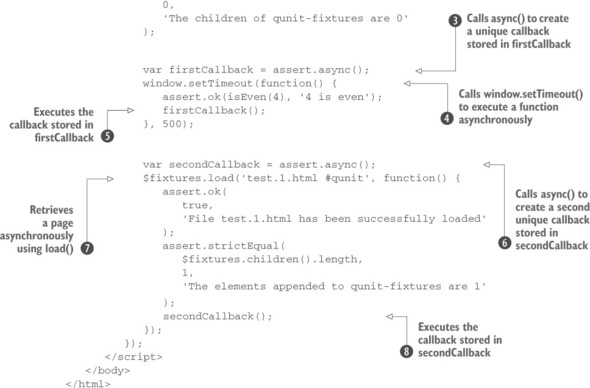
In this code you set up the markup to use QUnit and include jQuery. You also define the isEven() function to test if a number is even or not . Then, after including the QUnit test runner, you create an asynchronous test using the usual QUnit.test() method.
Inside the test you retrieve the element with the ID of qunit-feature that you’ll use to inject some elements later in the code. Then you set the number of asserts you expect to run and create a first assertion.
Next, you call the assert.async() method to create a first unique callback that’s stored in the variable named firstCallback . The first asynchronous operation you perform is to run a function with 500 milliseconds of delay by using the window .setTimeout() method
. Inside the callback defined, you test if 4 is even and execute the callback stored in firstCallback
. Executing this function is crucial because if you omit it, the test runner will wait indefinitely for the invocation of the function, blocking the execution of any other test.
In the last part of the code, you execute async() again to create a second unique callback . The second callback is stored in the variable secondCallback. The other asynchronous operation of the code uses jQuery’s load() method. You attempt to retrieve the file lesson-14/test.1.html and then inject only the element having qunit as its ID
. Inside the callback passed to load(), you verify that the file is correctly loaded using the assert.ok() method and that only one element has been injected into the element having as its ID qunit-fixture. Once this is finished, you execute the callback stored in secondCallback
.
Running asynchronous tests is easy, as you’ve seen in this example. The only aspect to remember is to invoke the callback functions generated using the async() method.
Sometimes when using jQuery’s Ajax functions you want to load resources from a server. It may happen that you’re developing the JavaScript code before the backend code has been written or that you don’t want to rely on the correctness of the backend code to ensure that your frontend code works as expected. For such situations, you can mock the Ajax requests using jQuery Mockjax or Sinon.js.
Earlier in this lesson we mentioned three check boxes on the user interface provided by QUnit when running tests. Let’s talk a bit more about the two of them that modify the behavior of QUnit.
Leave a Reply