In this last section you’ll build a complete test suite for a project, and what’s better than testing something you’ve developed in this very app? That’s why you’ll create a test suite for Jqia Context Menu, the jQuery plugin you built in lesson 12 to show a custom context menu on one or more specified elements of a page.
The first step needed to create the suite is to construct a new page with the essential setup for QUnit, where you’ll also need to include the jQuery library and the files relative to Jqia Context Menu (jquery.jqia.contextMenu.css and jquery.jqia.contextMenu.js). Once you’ve done so, you’ll place an unordered list that will act as the custom menu inside the element having qunit-fixture as its ID. You’ll also use this element as the one where you’ll show the custom menu.
You want to force yourself and all the people involved in writing the test suite to use the assert.expect() method. To do so you have to modify the default value of the QUnit configuration’s property requireExpects so that QUnit raises an error if it isn’t invoked.
The Jqia Context Menu plugin consists of one JavaScript file, so ideally you won’t need to group your tests into modules. But you must remove all the data attached to the element having the ID of qunit-fixture each time a test is completed. Therefore, you need to use afterEach as follows:
QUnit.module('Core', {
afterEach: function() {
$('#qunit-fixture').removeData();
}
});
After the declaration of the module you can define your tests. You’ll create five tests in total, divided as described here:
- Basic requirements —Contains the assertions that verify whether jQuery and the Jqia Context Menu plugin have been correctly loaded. In addition it checks that your plugin correctly exposes the default values to configure it.
- Wrong parameters —Defines the assertions to check that the plugin is able to deal with unexpected parameter types or missing mandatory properties (that is, idMenu).
- Initializations —Specifies the assertions to verify if the plugin breaks chainability and that it doesn’t raise errors when the right parameters are passed. In addition, it tests that the plugin can’t be initialized two or more times on the same element.
- Callbacks —Contains the assertions to test if the menu is displayed or hidden based on the events fired and what element caused the event.
- Destroy —Tests that the chainability is kept and that the method correctly removes all the data attached to the element. In addition, it verifies that the menu is hidden when the effect of the plugin is cancelled.
Coding the description of these tests results in the following listing.
Listing 14.4. The complete test suite for Jqia Context Menu
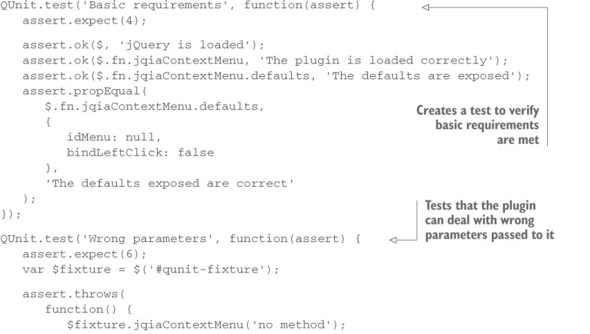
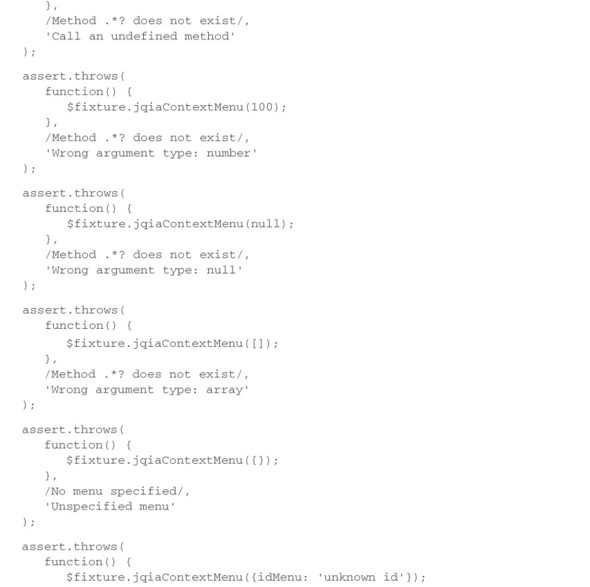
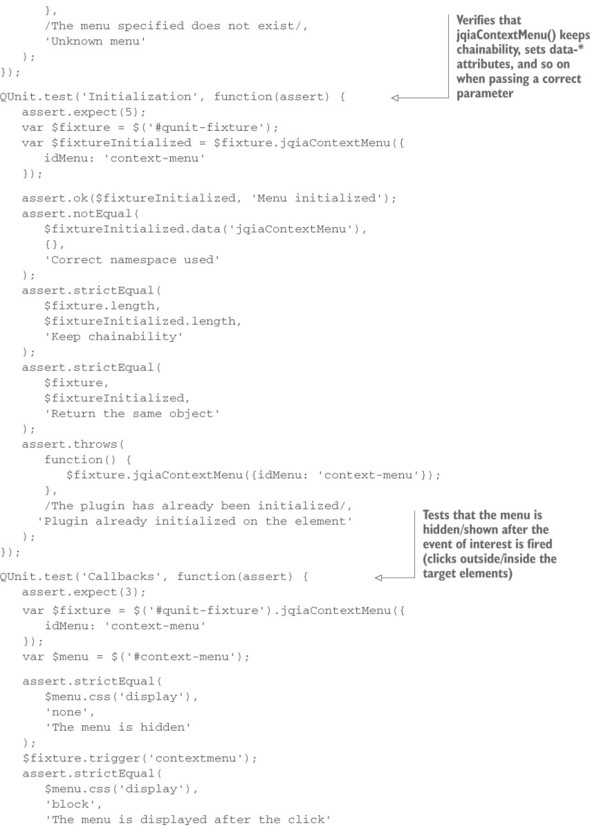
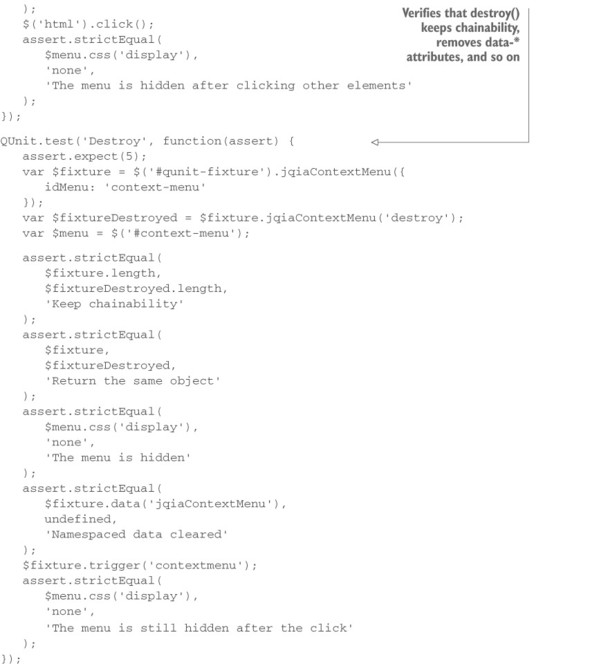
If you want to run this test suite and play with it a bit (maybe introducing other meaningful tests?), you’ll find it in the file lesson-14/test.suite.html.
This test suite has been created to pass all the tests, but to have a better grasp of the code written to test the plugin, you might want to see some tests failing. If you want to see some tests fail, try to change the code of the plugin in an unexpected way. If you need a suggestion, try to remove the return this; statement in the init() and the destroy() methods. Doing so will break the chainability of the plugin, and thus the relative assertions will fail.
This last demo showed you not only another example of most of the methods we covered in this lesson but also what a complete test suite looks like. We hope that you’ll take our advice to heart and start testing your code more frequently if you don’t do so already.
Leave a Reply