Human cognitive ability being what it is, making items pop into and out of existence instantaneously can be jarring. If you blink at the wrong moment, you could miss the transition, leaving you to wonder, “What just happened?”
Gradual transitions of a short duration help you know what’s changing and how you got from one state to the other—and that’s where the jQuery core effects come in. There are three sets of effect types:
- Show and hide (There’s a bit more to these methods than what we discussed in section 8.1.)
- Fade in and fade out
- Slide down and slide up
Let’s look more closely at each of these effect sets.
Showing and hiding elements gradually
The show(), hide(), and toggle() methods are more flexible than we led you to believe in the previous section. When called with no arguments, these methods effect a simple manipulation of the display state of the DOM elements, causing them to instantaneously be revealed or hidden. But when arguments are passed to them, these effects can be animated so that the changes in display status of the affected elements take place over a period of time.
With that, you’re now ready to look at the full syntaxes of these methods.
Method syntax: hide | |
---|---|
hide(duration[, easing][, callback]) hide(options) hide() | |
Causes the elements selected to become hidden. If called with no parameters, the operation takes place instantaneously by setting the display style property value of the elements to none. If a duration parameter is provided, the elements are hidden over a period of time by adjusting their width, height, and opacity downward to zero, at which time their display style property value is set to none to remove them from the display. | |
An optional easing function name can be passed to specify how the transition between the states is performed. An optional callback can be specified, and is invoked when the animation is complete. In its second version, you can provide an object containing some options to pass to the method (more on the properties available later). | |
Parameters | |
duration | (Number|String) Specifies the duration of the effect as a number of milliseconds or as one of the predefined value strings: “slow” (same as passing 600), “normal” (same as passing 400), or “fast” (same as passing 200). If this parameter is omitted and a callback function is specified as the first parameter, the value of “normal” is assumed. |
easing | (String) Specifies an easing function name to use when performing the transition from the visible state to the hidden state. The easing functions specify the pace of the animation at different points while in execution. If an animation takes place but this parameter isn’t specified, it defaults to “swing”. More about these functions in section 8.3. |
callback | (Function) A function invoked when the animation completes. No parameters are passed to this function, but the function context (this) is set to the element that was animated. The callback is fired for each element that undergoes animation. |
options | (Object) An optional set of options to pass to the method. The options available are shown in table 8.1. |
Returns | |
The jQuery collection. |
The hide() method gives us the opportunity to discuss several points. The first point is the easing parameter, which allows you to specify an easing function. The term easing is used to describe the manner in which the processing and pace of the frames of the animation are handled. By using some fancy math on the duration of the animation and current time position, some interesting variations to the effects are possible. But what functions are available? The jQuery core supports only two functions: linear, which progresses at a constant pace throughout the animation, and swing, which progresses slightly slower at the beginning and end of the animation than it does in the middle. “Why only two functions?” you may ask. The reason isn’t that jQuery wants to kill your creativity. Rather, jQuery wants to be as lean as possible by externalizing any additional feature to third-party libraries. You’ll learn how to add more easing functions (sometimes referred to as easings) in section 8.3.
Note
The value "normal" of the duration parameter is just a convention. You can use whatever string you like (except "slow" and "fast", of course) to specify a transition that has to last 400 milliseconds. For example, you may use "jQuery", "jQuery in Action", or "wow", obtaining exactly the same result. If you want to see it with your own eyes, search for the property jQuery.fx.speeds within the jQuery source. That said, we strongly advise you to stick with the usual "normal" value to avoid driving your colleagues crazy.
The other parameter worth a discussion is options. Using this parameter you can heavily customize how the hide() method acts. The properties and the values allowed are shown in table 8.1.
Table 8.1. The properties and the values, in alphabetic order, allowed in the options parameter
Property | Value |
---|---|
always | (Function) A function called when the animation completes or stops without completing. The Promise object passed to it is either resolved or rejected (we’ll discuss this concept in lesson 13). |
complete | (Function) A function called when the animation is completed. |
done | (Function) A function called when the animation completes, which means when its Promise object is resolved. |
duration | (String|Number) The duration of the effect as a number of milliseconds or as one of the predefined strings. Same as explained before. |
easing | (String) The function name to use when performing the transition from the visible state to the hidden state. Same as explained before. |
fail | (Function) A function invoked when the animation fails to complete. The Promise object passed to it is rejected. |
progress | (Function) A function executed after each step of the animation. The function is called only once per animated element regardless of the number of animated properties. |
queue | (Boolean|String) A Boolean specifying whether the animation has to be placed in the effects queue (more on this in a later section). If the value passed is false, the animation will begin immediately. The default value is true. In case a string is passed, the animation is added to the queue represented by that string. When a custom queue name is used, the animation does not automatically start. |
specialEasing | (Object) A map of one or more CSS properties whose values are easing functions. |
start | (Function) A function invoked when the animation begins. |
step | (Function) A function executed for each animated property of each animated element. |
All these properties enable you to create amazing effects. We’ll cover this topic shortly, developing three different custom effects. We know there are a lot of unclear concepts and you might be a bit confused. But don’t worry; everything will be explained in detail shortly, so please bear with us.
Now that you’ve studied in depth the hide() method and its parameters, you can learn more about show(). Because the meaning of the parameters is the same for show() as for hide(), we won’t repeat their description.
Method syntax: show |
---|
show(duration[, easing][, callback]) show(options) show() |
Causes any hidden elements in the set of matched elements to be revealed. If called with no parameters, the operation takes place instantaneously by setting the display style property value of the elements to an appropriate setting ( block or inline). If a duration parameter is provided, the elements are revealed over a specified duration by adjusting their width, height, and opacity upward to full size and opacity. An optional easing function name can be specified to define how the transition between the states is performed. An optional callback can be specified that’s invoked when the animation is complete. In its second version, you can provide an object containing some options to pass to the method, as described in table 8.1. |
Returns |
The jQuery collection. |
As you saw in our second example, jQuery provides a shortcut called toggle() to toggle the state of one or more elements. Its syntax is shown here, and in this case too we’ll omit the description of the parameters already described.
Method syntax: toggle | |
---|---|
toggle(duration[, easing][, callback]) toggle(options) toggle(condition) toggle() | |
Performs show() on any hidden element and hide() on any non-hidden element. See the syntax description of those methods for their semantics. In its third form, toggle() shows or hides the selected elements based on the evaluation of the passed condition. If true, the elements are shown; otherwise, they’re hidden. | |
Parameters | |
condition | (Boolean) Determines whether elements must be shown (if true) or hidden (if false). |
Returns | |
The jQuery collection. |
Let’s do a third take on the collapsible module, animating the opening and closing of the sections. Given the previous information about the toggle() method, you’d think that the only change you’d need to make to the code in listing 8.2 would be to change the call to toggle() to toggle('slow'). And you’d be right. But not so fast! Because that was just too easy, let’s take the opportunity to add an additional feature to the module.
Let’s say that to give the user an unmistakable visual clue, you want the module’s caption to display a different icon when it’s in its rolled-up state. You could make the change before firing off the animation, but it’d be much cooler to wait until the animation is finished.
jQuery 3: Feature changed
jQuery 3 changes the behavior of hide(), show(), toggle(), fadeIn(), and all the other related methods that we’ll cover in this lesson. All these methods will no longer override the CSS cascade. What this means is that if an element is hidden—because in your style sheet you have a declaration of display: none;—invoking show() (or similar methods like fadeIn() and slideDown()) on that element will no longer show it. To understand this change, consider the following element:
<div class="hidden">Hello!</div>
Now, let’s assume that in your style sheet you have the following code:
.hidden { display: none; }
If you’re using a version of jQuery prior to 3, if you write
$('div').show('slow');
you’ll see a nice animation that ultimately will show the element.
In jQuery 3, executing the same statement will have no effect as the library doesn’t override the CSS declaration. If you want to obtain the same result in jQuery 3, you should use a statement like the following instead:
$('div')
.removeClass('hidden')
.hide()
.show('slow');
Alternatively, you could use this statement:
$('div')
.removeClass('hidden')
.css('display', 'none')
.show('slow');
This change is controversial and will probably break the code of many websites. At the time of writing, the final version of jQuery 3 has not been published, so this new behavior could be modified or completely reverted. Please take the time to check the official documentation to learn more.
You can’t just make the call right after the animation method call because animations don’t block. The statements following the animated method call would execute immediately, probably even before the animation has had a chance to commence. This is a great occasion to use the callback parameter of toggle().
The approach you’ll take is that after the animation is complete, you’ll replace the text of the <span> containing the icon. You’ll use the minus sign if the body is visible (to indicate that it can be collapsed) and the plus sign if the body is hidden (to indicate that it can be expanded). You could also have done this by working with CSS and the content property, by adding a class name to the module to indicate that it’s rolled up and removing the class name otherwise. But because this isn’t a app on CSS, we’ll skip this solution. The next listing shows the change that you need to make to your code to make it happen.
Listing 8.3. Animated version of the module, with a change of the caption icon
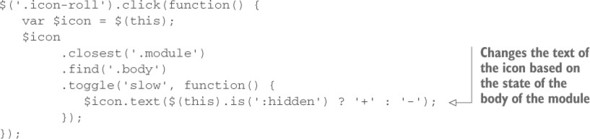
You can find the page with these changes in file lesson-8/collapsible.module.take .3.html.
Knowing how much people love to tinker, we’ve set up a handy tool that we’ll use to further examine the operation of these and the remaining effect methods.
Leave a Reply