As we discussed in the introduction, when working on large projects there’s a real need to have good code organization. Imagine for a moment what would happen to software developed by companies like Google or Microsoft if they had bad code organization. With products where new features are added and old ones are updated frequently, poorly structured code leads to an incredible amount of time wasted. And as you know, time is money.
One of the most used patterns to structure software is the Model–View–Controller (MVC) pattern. It’s a software architectural pattern that separates concerns into three main concepts: model, view, and controller. The model represents the application data, such as a registered user of a website. The view is the component that deals with displaying the data—that is, how the data will be shown in the web page if you’re using this pattern on the web. The controller updates the model’s state and sends the data to the view (for example, a change in the address of the user).
If you were a PHP developer, you’d be aware of Symphony, Laravel, or Zend Framework; if you were a Java developer, you’d probably have heard of Spring Web MVC or Struts. But because we’re talking about JavaScript, we have other frameworks. One of these frameworks that implements the MVC pattern is Backbone.js.
Ideally, jQuery could be used to create single-page applications, but because that isn’t its main purpose, more often than not you’ll end up with complex, hard-to-write, and hard-to-maintain code. To create such applications, you need a framework specifically developed with this scope in mind, such as Backbone.js. Backbone.js has some libraries on which it relies: Underscore.js and jQuery. Therefore, the knowledge you’ve acquired so far will be useful even in this context.
Today many web applications rely on JavaScript and Ajax to create better user interactions and to create single-page applications (SPAs). These are applications that, once loaded in the browser, perform all the HTTP requests without requiring the whole page to reload. In terms of performance, this is a big advantage because the browser doesn’t have to load all the assets (JavaScript files, CSS files, fonts, and so on) again. It loads only the small portion that has to be injected into the DOM, returned by the server.
The presented approach doesn’t come without cost. SPAs usually have a longer loading time because they require loading more code than other applications. For this reason, you have to pay attention to balancing the code needed by your application and its weight. A page that takes too long to load is at risk of losing users.
In the following sections, we’ll discuss the features of the pattern implemented by the framework and also give a brief overview of its main concepts such as models, views, and routers. In addition, we’ll develop a simple yet useful application to organize to-do lists (from now on we’ll refer to it as the Todos manager). We chose this application because it’s often used as a project when learning a new framework. Its scope is to save the tasks you have to do so you can easily remember them. Please note that this section isn’t intended to be an in-depth guide to Backbone.js, and we’ll only skim the surface. If you want a complete resource, you can buy a app dedicated to this framework.
Why use an MV* framework?
Just like a lot of things in life, programming is a cycle. You have a problem that you solve using the tools you have at a given moment, but then new libraries and frameworks are released to improve the code and the solutions adopted. Then new issues arise and the cycle starts again.
When the first SPAs came out, many developers started to employ jQuery (or similar libraries) and a lot of callbacks and Ajax calls to synchronize the user input with the data stored on the server. Once these applications increased in complexity, the code became unmaintainable and unscalable. As a consequence, developers needed a framework to help them with the development of a well-structured and maintainable code. That’s where frameworks like Backbone.js, AngularJS, Ember, and many others came into play. They’re usually referred to as MV* frameworks because they don’t quite fit either the MVC pattern, the MVP (Model-View-Presenter) pattern, or the MVVM (Model-View-ViewModel) pattern.
An illustration representing the MVC pattern and the Backbone.js implementation of this pattern is shown in figure 15.3.
Figure 15.3. The MVC pattern compared to the Backbone.js implementation
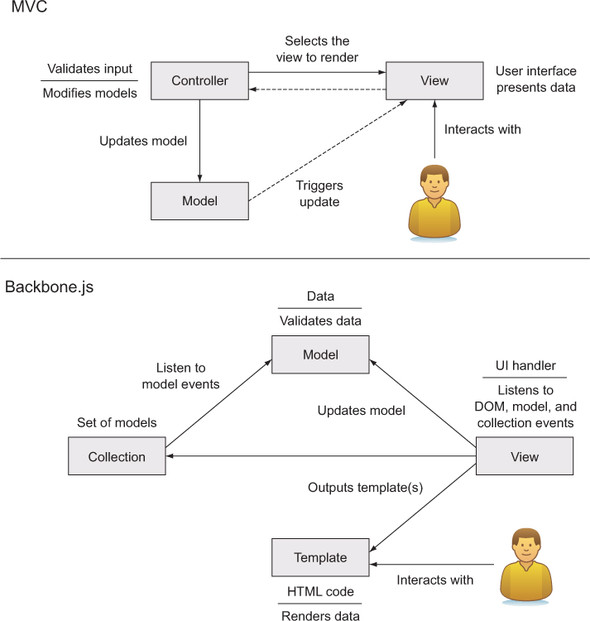
As you can see from figure 15.3, the main difference between the classic MVC pattern and the Backbone.js implementation lies in the view component. The view also acts as a controller, handling the rendering of the UI that’s represented by the template block, and has the responsibility of updating the model.
Now that you’re aware of the model implemented by Backbone.js, let’s delve into each component, one at a time, to analyze its responsibilities and features.
Leave a Reply