Queuing up functions for execution is not all that useful unless you can somehow cause the execution of the functions to actually occur. Enter the dequeue() method.
Method syntax: dequeue | |
---|---|
dequeue([name]) | |
Removes the foremost function in the named queue for each element in the jQuery object and executes it for each element. | |
Parameters | |
name | (String) The name of the queue from which the foremost function is to be removed and executed. If omitted, the default effects queue of fx is assumed. |
Returns | |
The jQuery collection |
When dequeue() is invoked, the foremost function in the named queue for each element in the jQuery object (the first in the queue) is executed, with the function context for the invocation (this) being set to the element. Consider the code in the following listing, available in the file lesson-8/manual.dequeue.html.
Listing 8.8. Queuing and dequeuing functions on multiple elements
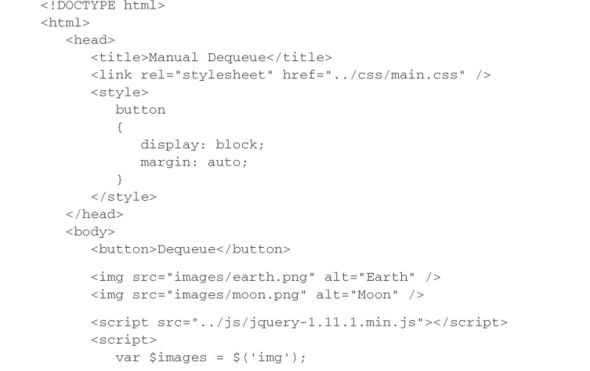
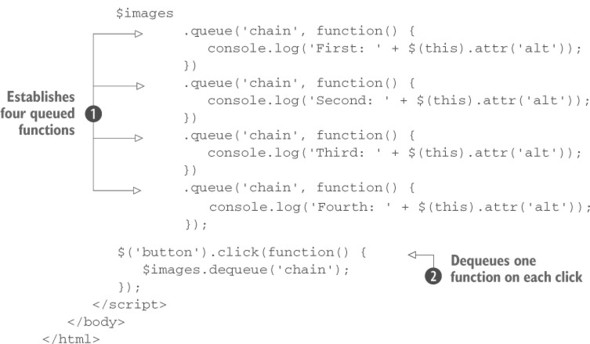
In this example, you have two images upon which you add functions to a queue named chain . Inside each function you emit on the console the alt attribute of whatever DOM element is serving as the function context and a number relating to its position in the queue. This way, you can tell which function is being executed and from which element’s queue. So, at the moment, you aren’t doing anything special with these images (they won’t move). Upon clicking the Dequeue button, the button’s click handler
causes a single execution of the dequeue() method. Go ahead and click the button once and observe the messages in the console, as shown in figure 8.9.
You can see that the first function you added to the chain queue for the images has been fired twice: once for the Earth image and once for the Moon image. Clicking the Dequeue button more times removes the subsequent functions from the queues one at a time and executes them until the queues have been emptied, after which calling dequeue() has no effect.
In this example, the dequeuing of the functions was under manual control—you needed to click the button four times (resulting in four calls to dequeue()) to get all four functions executed. Frequently you want to trigger the execution of the entire set of queued functions. For such times, a commonly used idiom is to call the dequeue() method within the queued function in order or using the next parameter passed to the queued function. Using one of these two techniques, you trigger the execution of the next queued function, creating a sort of chain of calls.
Figure 8.8. Clicking the Dequeue button causes a single queued instance of the function to fire, once for each image that it was established upon.
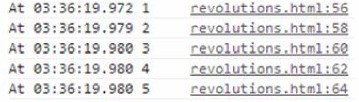
Consider the following changes to the code in listing 8.8 that uses both techniques mentioned:
var $images = $('img');
$images
.queue('chain', function(next) {
console.log('First: ' + $(this).attr('alt'));
next();
})
.queue('chain', function(next) {
console.log('Second: ' + $(this).attr('alt'));
next();
})
.queue('chain', function() {
console.log('Third: ' + $(this).attr('alt'));
$(this).dequeue('chain');
})
.queue('chain', function() {
console.log('Fourth: ' + $(this).attr('alt'));
});
The modified version of the file lesson-8/manual.dequeue.html that includes the previous snippet can be found in the file lesson-8/automatic.dequeue.html.
Bring up that page in your browser and click the Dequeue button. Note how the single click now triggers the execution of the entire chain of queued functions, as shown by figure 8.10. Also note that the last function added to the queue doesn’t have a call to dequeue() because at that time the queue will already be empty.
With dequeue() you can execute the function at the front of the queue. But sometimes you may need to remove all the functions stored in the queue without executing them.
Figure 8.9. Clicking the Dequeue button causes all the functions in the queue to fire for every image that they were established upon.
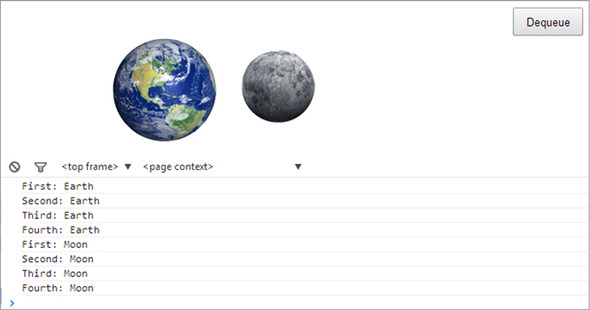
Clearing out unexecuted queued functions
If you want to remove the queued functions from a queue without executing them, you can do that with the clearQueue() method.
Method syntax: clearQueue | |
---|---|
clearQueue([name]) | |
Removes all unexecuted functions from the named queue. | |
Parameters | |
name | (String) The name of the queue from which the functions are to be removed without execution. If omitted, the default effects queue of fx is assumed. |
Returns | |
The jQuery collection |
Although similar to the stop() animation method, clearQueue() is intended for use on general queued functions rather than just animation effects.
Sometimes, instead of clearing the queue you may need to delay the execution of a queue function. Let’s discuss this possibility.
Leave a Reply