When it comes to CSS styles that you want to set or get on your pages, is there a more common set of properties than the element’s width or height? Probably not, so jQuery makes it easy for you to deal with the dimensions of the elements as numeric values rather than strings.
Specifically, you can get (or set) the width and height of an element as a number by using the convenient width() and height() methods. You can set the width or height as follows.
Method syntax: width and height | |
---|---|
width(value) height(value) | |
Sets the width or height of all elements in the matched set. | |
Parameters | |
value | (Number|String|Function) The value to be set. This can be a number of pixels or a string specifying a value in units (such as px, em, or %). If no unit is specified, px is the default. If a function is provided, the function is invoked for each element in the set, passing that element as the function context (this). The function is passed two values: the element index and the element’s current value. The function’s returned value is used as the new value. |
Returns | |
The jQuery collection. |
Keep in mind that these are shortcuts for the css() method, so
$('div').width(500);
is identical to
$('div').css('width', 500);
You can also retrieve the width or height as follows.
Method syntax: width and height | |
---|---|
width() height() | |
Retrieves the width or height of the first element of the jQuery object | |
Parameters | |
none | |
Returns | |
The computed width or height as a number in pixels; null if the jQuery object is empty |
The getter version of these two methods is a bit different from its css() counterpart. css() returns a string containing the value and the unit measure (for example, "40px"), whereas width() and height() return a number, which is the value without the unit and converted into a Number data type. If your style defines the width or height using units different from pixels (em, %, and so on), jQuery will still return the value relative to the width or height of the element in pixels.
jQuery 3: Bug fixed
jQuery 3 fixes a bug of the width(), height(), and all the other related methods. These methods will no longer round to the nearest pixel, which made it hard to position elements in some situations. To understand the problem, let’s say that you have three elements with a width of 33% inside of a container element that has a width of 100px:
<div class="wrapper">
<div>Hello</div>
<div>Hi</div>
<div>Bye</div>
</div>
Prior to jQuery 3, if you tried to retrieve the width of one of the three children elements as follows
$('.wrapper div:first').width();
you’d obtain the value 33 as the result because jQuery rounds the value 33.33333. In jQuery 3 this bug has been fixed, so you’ll obtain more accurate results.
The fact that the width and height values are returned from these functions as numbers isn’t the only convenience that these methods bring to the table. If you’ve ever tried to find the width or height of an element by looking at its style.width or style.height properties, you were confronted with the sad truth that these properties are only set by the corresponding style attribute of that element; to find out the dimensions of an element via these properties, you have to set them in the first place. Not exactly a paragon of usefulness!
The width() and height() methods, on the other hand, compute and return the size of the element. Knowing the precise dimensions of an element in simple pages that let their elements lay out wherever they end up isn’t usually necessary, but knowing such dimensions in highly interactive scripted pages is crucial to being able to correctly place active elements, such as context menus, custom tool tips, extended controls, and other dynamic components.
Let’s put them to work. Figure 5.2 shows a sample page that was set up with two primary elements: a div serving as a test subject that contains a paragraph of text (with a border and background color for emphasis) and a second div in which to display the dimensions. To write the dimensions in the second div, we’ll use the html() method that we’ll cover shortly.
Figure 5.2. The width and height of the test element aren’t fixed and depend on the width of the browser window.
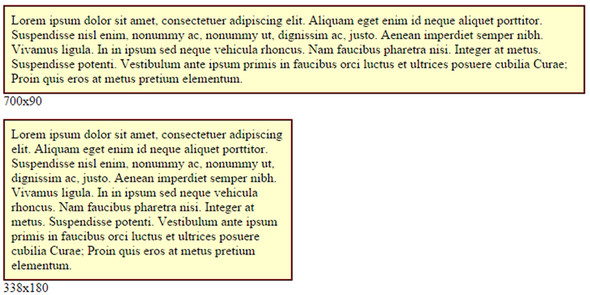
The dimensions of the test subject aren’t known in advance because no style rules specifying dimensions are applied. The width of the element is determined by the width of the browser window, and its height depends on how much room will be needed to display the contained text. Resizing the browser window will cause both dimensions to change.
In our page, we define a function that will use the width() and height() methods to obtain the dimensions of the test subject div (identified as test-subject) and display the resulting values in the second div (identified as display):
function displayDimensions() {
$('#display').html(
$('#test-subject').width() + 'x' + $('#test-subject').height()
);
}
We call this function as the last statement of our script, resulting in the initial values being displayed, as shown in the upper portion of figure 5.2.
We also add a call to the same function in a resize handler on the window that updates the display whenever the browser window is resized (you’ll learn how to do this in lesson 6), as shown in the lower portion of figure 5.2. The full code of this page is shown in the following listing and can be found in the file lesson-5/dimensions.html.
Listing 5.1. Dynamically tracking and displaying the dimensions of an element
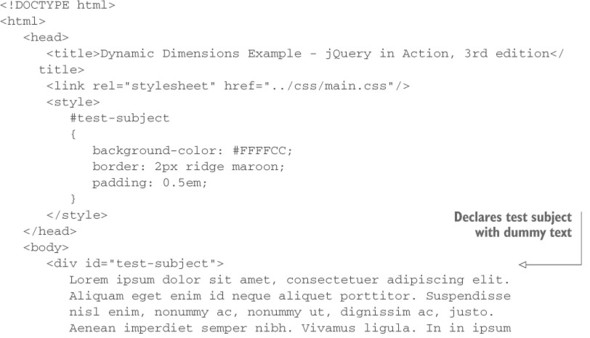
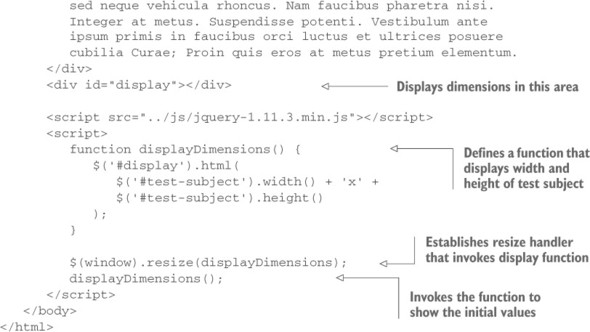
In addition to the convenient width() and height() methods, jQuery also provides similar methods for getting more particular dimension values, as described in table 5.1.
Table 5.1. Additional jQuery dimension-related methods
Method | Description |
---|---|
innerHeight() | Returns the inner height of the first matched element, which excludes the border but includes the padding. The value returned is of type Number unless the jQuery object is empty, in which case null is returned. If a number is returned, it refers to the value of the inner height in pixels. |
innerHeight(value) | Sets the inner height of all the matched elements with the value specified by value. The type of value can be String, Number, or Function. The default unit used is px. If a function is provided, it’s called for every element in the jQuery object. The function is passed two values: the index position of the element within the jQuery object and the current inner height value. Within the function, this refers to the current element within the jQuery object. The returned value of the function is set as the new value of the inner height of the current element. |
innerWidth() | Same as innerHeight()except it returns the inner width of the first matched element, which excludes the border but includes the padding. |
innerWidth(value) | Same as innerHeight(value) except the value is used to set the inner width of all the matched elements. |
outerHeight ([includeMargin]) | Same as innerHeight() except it returns the outer height of the first matched element, which includes the border and the padding. The includeMargin parameter causes the margin to be included if it’s true. |
outerHeight(value) | Same as innerHeight(value) except the value is used to set the outer height of all the matched elements. |
outerWidth ([includeMargin]) | Same as innerHeight() except it returns the outer width of the first matched element, which includes the border and the padding. The includeMargin parameter causes the margin to be included if it’s true. |
outerWidth(value) | Same as innerHeight(value) except the value is used to set the outer width of all the matched elements. |
You’re not finished yet; jQuery also gives you easy support for positions and scrolling values.
Leave a Reply