The backend of your project has two main responsibilities: validating the user’s input and sending the email. The former is the most interesting one for your purposes because, based on the specifications of your project, you have to deal with two different cases. The first case is a partial request resulting from the loss of the focus of a field. The second is a request containing the values of all the fields, resulting from the click of the Submit button.
To distinguish between these two cases, you’ll add a custom parameter called partial to your partial requests (more on this when we discuss the JavaScript code). In such situations, the PHP page has to skip all the validations but the one related to the field that has lost the focus. Then it has to return a JSON object according to the result of the test. The result will be stored in a variable called $result (we have such an imagination!) and returned (using the echo language construct) using a PHP function called json_encode() as shown here:
echo json_encode($result);
To distinguish between the partial and the complete request, you’ll employ a variable called $isPartial whose value is set as follows:
$isPartial = empty($_POST['partial']) ? false : true;
To help you understand the backend code, let’s analyze the part of the code relative to the name of the user, shown in the next listing.
Listing 11.2. The code for the name field test
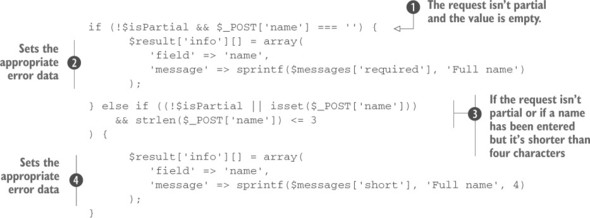
The most interesting parts of this listing are the two if conditions. In the first one, you test if the current request isn’t partial (negating the $isPartial variable) and the value is empty ($_POST['name'] === '') . If both evaluate to true, you set the appropriate error message specifying that the value is mandatory and thus must be filled
.
The second if is a bit trickier. You want to verify whether the value of the field is shorter than four characters (strlen($_POST['name']) <= 3) and if so, return an error. But it’s when you want to perform the validation that things get interesting. You have to verify the length if the request isn’t partial (!$isPartial), so all the fields must be validated, or if the request is partial and it concerns the name field ($isPartial && isset($_POST['name'])). Based on this discussion, you might think that the final condition to use is this:
!$isPartial || ($isPartial && isset($_POST['name']))
But the part on the right of the OR operator will only be evaluated if the part on the left is false—that is, when the request is partial. Relying on this information, you can shorten the condition, obtaining the following:
!$isPartial || isset($_POST['name'])
If the condition evaluates to true, you set the appropriate error message .
Understanding this condition may be hard at first, but reading the code carefully a couple of times should prove that we wrote the code properly. In case you’re still unsure, you can take a look at the diagram in figure 11.4.
Figure 11.4. The process used to validate the user’s input
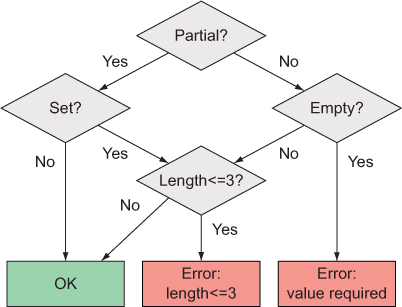
The validation of the other fields is similar to the one we just described, so we’ll omit their discussion.
Now that we’ve covered the backend code, it’s time to delve into the most interesting part of our project: the JavaScript code. In the next section you’ll discover how you can employ jQuery to tie together the pieces you’ve built in order to bring your page to life.
Leave a Reply