Now that you know what jQuery is and what it can do for you, you need to download the library to start getting your hands dirty. Once there, you’ll probably be overwhelmed by the plethora of options available. Branch 1.x, 2.x, or 3.x? Compressed or uncompressed? Download it or use a content delivery network (CDN)? Which one to choose depends on several factors. To make a conscious choice, let’s uncover the differences.
Choosing the right version
In April 2013, the jQuery team introduced version 2.0 with the intention of looking at the future of the web instead of its past, especially from the browser’s perspective. Until that point, jQuery supported all of the latest versions of Chrome, Firefox, Safari, Opera, and Internet Explorer starting from version 6. With the introduction of version 2.0, the team decided to leave behind the older Internet Explorer 6, 7, and 8 browsers to focus on the web as it will be, not as it was.
This decision caused the deletion of a bunch of code created to solve browser incompatibilities and missing features in those prehistoric browsers. The fulfillment of this task resulted in a smaller (-12%) and faster code base. Although 1.x and 2.x are two different branches, they have a strict relation. There’s feature parity between jQuery version 1.10 and 2.0, version 1.11 and 2.1, and so on.
In October 2014, Dave Methvin, the president of the jQuery Foundation, published a blog post where he publicly announced the plan to release a new major version of jQuery: jQuery 3. In the same way version 1.x supports old browsers while 2.x targets modern browsers, jQuery 3 is split into two versions. jQuery Compat 3 is the successor of 1.x, whereas jQuery 3 is the successor of 2.x. He further explained:
We’ll also be re-aligning our policy for browser support starting with these releases. The main jQuery package remains small and tight by supporting the evergreen browsers (the current and previous versions of a specific browser) that are common at the time of its release. We may support additional browsers in this package based on market share. The jQuery Compat package offers much wider browser support, but at the expense of a larger file size and potentially lower performance.
With the new version, the team also took the opportunity to drop the support for some browsers, fix many bugs, and improve several features.
The first factor to consider when deciding which version to use is which browsers your project must support. Table 1.1 describes the browsers supported by each major version of jQuery.
Table 1.1. An overview of the browsers supported by the major versions of jQuery
Browsers | jQuery 1 | jQuery 2 | jQuery Compat 3 | jQuery 3 |
---|---|---|---|---|
Internet Explorer | 6+ | 9+ | 8+ | 9+ |
Chrome | Current and previous | Current and previous | Current and previous | Current and previous |
Firefox | Current and previous | Current and previous | Current and previous | Current and previous |
Safari | 5.1+ | 5.1+ | 7.0+ | 7.0+ |
Opera | 12.1x Current and previous | 12.1x Current and previous | Current and previous | Current and previous |
iOS | 6.1+ | 6.1+ | 7.0+ | 7.0+ |
Android | 2.3 4.0+ | 2.3 4.0+ | 2.3 4.0+ | 2.3 4.0+ |
As you can see from the table, there’s a certain degree of overlap in regard to the browser versions supported. But keep in mind that what’s referred to as “Current and previous” (meaning the current and preceding version of a browser at the time a new version of jQuery is released) changes based on the release date of the new version of jQuery.
Another important factor to base your decision on is where you’ll use jQuery. Here are some use cases that can help you in your choice:
- Websites that don’t need to support older versions of Internet Explorer, Opera, and other browsers can use branch 3.x. This is the case for websites running in a controlled environment such as a company local network.
- Websites that need to target an audience as wide as possible, such as a government website, should use branch 1.x.
- If you’re developing a website that needs to be compatible with a wider audience but you don’t have to support Internet Explorer 6–7 and old versions of Opera and Safari, you should use jQuery Compat 3.x.
- If you don’t need to support Internet Explorer 8 and below, but you have to support old versions of Opera and Safari, you should use jQuery 2.x.
- Mobile apps developed using PhoneGap or similar frameworks can use jQuery 3.x.
- Firefox OS or Chrome OS apps can use jQuery 3.x.
- Websites that rely on very old plugins, depending on the actual code of the plugins, may be forced to use jQuery 1.x.
In summary, two of the factors are where you’re going to use the library and which browsers you intend to support.
Another source of confusion could be the choice between the compressed (also referred to as minified) version, intended for the production stage, or the uncompressed version, intended for the development stage (see the comparison in figure 1.3). The advantage of the minified library is the reduction in size that leads to bandwidth savings for the end users. This reduction is achieved by removing the useless spaces (indentation), removing the code’s comments that are useful for developers but ignored by the JavaScript engines, and shrinking the names of the variables (obfuscation). These changes produce code that’s harder to read and debug—which is why you shouldn’t use this version in development—but smaller in size.
Figure 1.3. At the top, a snippet taken from the jQuery’s source code that shows you the uncompressed version format. At the bottom, the same snippet minified to be used in production.
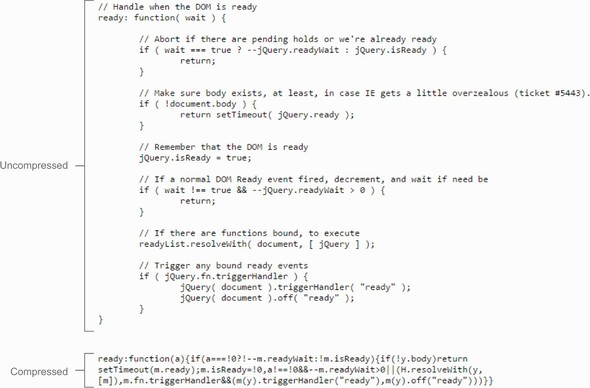
In this app we’ll use jQuery 1.x as a base to let you test your code in the widest range of possible browsers, but we’ll highlight all the differences introduced by jQuery 3 so that your knowledge will be as up to date as possible.
Choosing the right version of jQuery is important, but we also cited the difference between hosting jQuery locally or using a CDN.
Improving performances using a CDN
Today it’s common practice to serve files like images and libraries through a content delivery network to improve the performance of a website. A CDN is a distributed system of servers created to offer content with high availability and performance. You might be aware that browsers can download a fixed set of contents, usually from four to eight files, from a host at the same time. Because the files served using a CDN are provided from a different host, you can speed up the whole loading process, increasing the number of files downloaded at a time. Besides, a lot of today’s websites use CDNs, so there’s a higher probability that the required library is already in the user’s browser cache. Employing a CDN to load jQuery doesn’t guarantee better performance in every situation because there are many factors that come into play. Our advice is to test which configuration best suits your specific case.
Nowadays there are several CDNs you can rely on to include jQuery, but the most reliable are the jQuery CDN, the Google CDN, and the Microsoft CDN.
Let’s say you want to include the compressed version of jQuery 1.11.3 using the jQuery CDN. You can do that by writing the following code:
<script src="//code.jquery.com/jquery-1.11.3.min.js"></script>
As you may have noticed, this code doesn’t specify the protocol to use (either HTTP or HTTPS). Instead, you’re specifying the same protocol used in your website. But keep in mind that using this technique in a page that doesn’t run on a web server will cause an error.
Using a CDN isn’t all wine and roses, though. No server or network has 100% uptime on the internet, and CDNs are no exception. If you rely on a CDN to load jQuery, in the rare situations where it’s down or not accessible and the visitor’s browser doesn’t have a cached copy, your website’s code will stop working. For critical applications this can be a real problem. To avoid it, there’s a simple and smart solution you can adopt, employed by a lot of developers. Once again, you want to include the minified version of jQuery 1.11.3, but now you’ll use this smart solution:
<script src="//code.jquery.com/jquery-1.11.3.min.js"></script>
<script>window.jQuery || document.write('<script src="javascript/jquery-
1.11.3.min.js"><\/script>');</script>
The idea behind this code is to request a copy of the library from a CDN and check if it has been loaded, testing whether the jQuery property of the window object is defined. If the test fails, you inject a code that will load a local hosted copy that, in this specific example, is stored in a folder called javascript. If the jQuery property is present, you can use jQuery’s methods safely without the need to load the local hosted copy.
You test for the presence of the jQuery property because, once loaded, the library adds this property. In it you can find all the methods and properties of the library. During the development process, we suggest that you use a local copy of jQuery to avoid any connectivity problems.
In addition to the jQuery property, you’ll also find a shortcut called $ that you’ll see a lot in the wild and in this app. Although it may seems odd, in JavaScript a variable or a property called $ is allowed. We called $ a shortcut because it’s actually the same object of jQuery as proved by this statement taken from the source code:
window.jQuery = window.$ = jQuery;
So far, you’ve learned how to include jQuery in your web pages but you know nothing about how it’s structured. We’ll look at this topic in the next section.
Leave a Reply