Once you have a jQuery set, whether identified from existing DOM elements with selectors or created as new elements using HTML snippets (or a combination of both), you’re ready to manipulate those elements using the powerful set of jQuery methods. We’ll start looking at those methods in the next lesson, but what if you want to further refine the jQuery set? In this section, we’ll explore the many ways that you can refine, extend, or filter the jQuery set that you wish to operate upon.
In order to help you in this endeavor, we’ve included another lab in the downloadable project code for this lesson: the jQuery Operations Lab Page (lesson-3/lab.operations.html). This page, which looks a lot like the Selectors Lab we employed in lesson 2, is shown in figure 3.3.
Figure 3.3. The jQuery Operations Lab Page lets you compose jQuery collections in real time to help you see how collections can be created and managed.
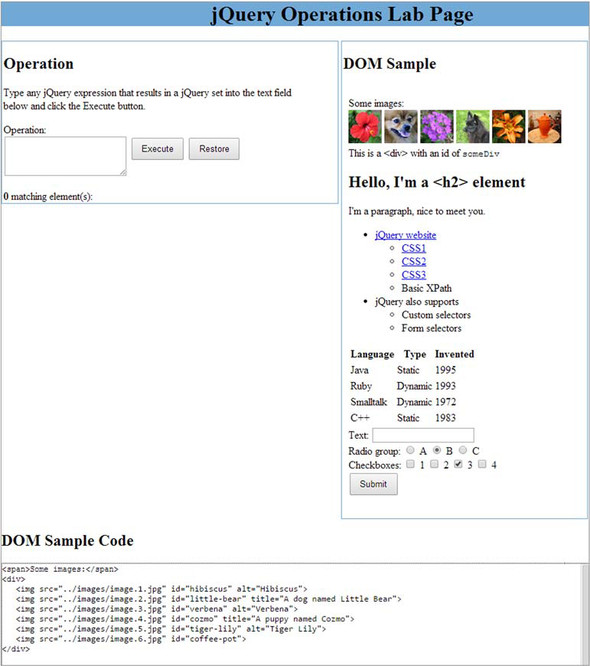

This new lab page not only looks like the Selectors Lab, but it also operates in a similar fashion. But in this lab, rather than typing a selector, you can type in any complete jQuery operation that results in a jQuery collection. The operation is executed in the context of the DOM Sample, and, as with the Selectors Lab, the results are displayed.
Note
This lab page loads the elements upon which it acts inside an iframe. Due to the security restrictions of some browsers, this operation may fail. To avoid this issue, you can either execute the page under a web server like Apache, Tomcat, or IIS or search for a specific solution for your browser. For example, in WebKit-based browsers, you can run them through the command-line interface (CLI) using the flag --allow
-file
-access
-from
-files. It’s important that the command creates a new process, so it must not open a new tab but a new window.
The jQuery Operations Lab allows you to enter any expression that results in a jQuery set. Because of the way jQuery chaining works, this expression can also include jQuery methods, making this a powerful lab for examining the operations of jQuery.
Be aware that you need to enter valid syntax, as well as expressions that result in a jQuery set. Otherwise, you’re going to be faced with a handful of unhelpful JavaScript errors.

To get a feel for the lab, load it in your browser and enter this text into the Operation field:
$('img').hide();
Then click the Execute button. This operation is executed within the context of the DOM Sample, and you’ll see how the images disappear from the sample.
After any operation, you can restore the DOM Sample to its original condition by clicking the Restore button. Although we haven’t treated it yet, the hide() method belongs to jQuery, and you’ll see it in detail later in this app. For the moment, what you need to know is that this function allows you to hide all the elements in a set. We used it because we wanted to give you a concrete example of what you can do in the Operations Lab Page. You’ll see this new lab in action as you work your way through the sections that follow, and you might even find it helpful in later lessons to test various jQuery operations.
Determining the size of a set
We mentioned before that a jQuery set acts a lot like an array. This mimicry includes a length property, just like JavaScript arrays, that contains the number of elements in the jQuery collection.
Let’s say you want to know the number of all the paragraphs in your page and show it on the screen; you can write the following statement:
alert($('p').length);
Okay, so now you know how many elements you have. What if you want to access them directly?
Obtaining elements from a set
Once you have a jQuery set, you often use jQuery methods to perform some sort of operation upon it as a whole. There may be times when you want a direct reference to an element or elements to perform raw JavaScript operations upon them. Let’s look at some of the ways that jQuery allows you to do just that.
Fetching elements by index
Because jQuery allows you to treat a jQuery collection as a JavaScript array, you can use simple array indexing to obtain any element in the list by position. For example, to obtain the first element in the set of all <img>s with an alt attribute on the page, you could write
var imgElement = $('img[alt]')[0];
The most observant of you might have noticed that we didn’t prepend the dollar sign ($) in front of the variable name (imgElement). We didn’t forget it. This jQuery set contains an array of DOM elements, so if you retrieve a single element, it isn’t a jQuery set made of one element itself but a plain DOM element.
If you prefer to use a method rather than array indexing, jQuery defines the get() method for that purpose.
Method syntax: get | |
---|---|
get([index]) | |
Obtains one or all of the matched elements in the set. If no parameter is specified, all elements in the jQuery object are returned as a JavaScript array. If an index parameter is provided, the indexed element is returned. index can be negative, in which case the count is performed starting from the end of the matched set. | |
Parameters | |
index | (Number) The index of the single element to return. If omitted, the entire set is returned as an array. If a negative integer is given, the count starts from the end of the set. If the index is out of bounds, which is less than the negative number of elements or equal to or greater than the number of elements, the method returns undefined. |
Returns | |
A DOM element, or an array of DOM elements, or undefined. |
The fragment
var imgElement = $('img[alt]').get(0);
is equivalent to the previous example that used array indexing.
The get() method also accepts a negative index. Using get(-1) will retrieve the last element in the set, get(-2) the second to last, and so on. In addition to obtaining a single element, get() can also return an array of all the elements of the set if used without a parameter.
Sometimes you’ll want a jQuery object containing a specific element rather than the plain element itself. It would look weird (although valid) to write something like this:
$($('p').get(2))
For this purpose, jQuery provides the eq() method. The latter mimics the action of the :eq() selector filter discussed in the previous lesson. To see their differences in terms of code, let’s say you want to select the second element in a set containing all the <div>s of a page. Here’s how you can perform this task, reporting the alternatives side by side:

The difference between the statements is minimal, but for performance reasons (more details in lesson 15) it’s better to stick with the first form (the eq() method). As a rule of thumb, we suggest you use methods over filters because they usually lead to better performance.
Now that we’ve highlighted the difference between the method and the filter, it’s time to dive into the details of the former.
Method syntax: eq | |
---|---|
eq(index) | |
Obtains the indexed element in the set and returns a new set containing just that element. | |
Parameters | |
index | (Number) The index of the single element to return. A negative index can be specified to select the element starting from the end of the set. |
Returns | |
A jQuery collection containing one or zero elements. |
Obtaining the first element of a set is such a common operation that there’s a convenience method that makes it even easier: the first() method.
Method syntax: first |
---|
first() |
Obtains the first element in the set and returns a new set containing just that element. If the original set is empty, so is the returned set. |
Parameters |
none |
Returns |
A jQuery collection containing one or zero elements. |
The first() method has its filter counterpart in the :first filter. Once again, we want to show an example of the two alternatives. Say that you want to retrieve the first paragraph of the page; you can write one of the following:

Not surprisingly, the difference in terms of code is minimal, but the first() method should be preferred to the :first filter.
As you might expect, there’s a corresponding method to obtain the last element in a set as well, which is the counterpart of the :last filter.
Method syntax: last |
---|
last() |
Obtains the last element in the set and returns a new set containing just that element. If the original set is empty, so is the returned set. |
Parameters |
None |
Returns |
A jQuery collection containing one or zero elements. |
If you want to practice with these methods, you can use the jQuery Operations Lab Page. For example, if you want to retrieve the first list item of the list shown in the page, you can write

$('li', '.my-list').first();
Now let’s examine the other method to obtain an array of elements in the set.
Fetching all the elements as an array
If you wish to obtain all of the elements in a jQuery object as a JavaScript array of DOM elements, jQuery provides the toArray() method.
Method syntax: toArray |
---|
toArray() |
Returns the elements in the set as an array of DOM elements. |
Parameters |
None |
Returns |
A JavaScript array of the DOM elements within the sets. |
Consider this example:
var allLabeledButtons = $('label + button').toArray();
This statement collects all the <button>s on the page that are immediately preceded by <label>s into a jQuery object and then creates a JavaScript array of those elements to assign to the allLabeledButtons variable.
Finding the index of an element
Whereas get() finds an element given an index, you can use an inverse operation, index(), to find the index of a particular element in the set. The syntax of the index() method is as follows.
Method syntax: index | |
---|---|
index([element]) | |
Finds the specified element in the set and returns its ordinal index within the set, or finds the ordinal index of the first element of the set within its siblings. If the element isn’t found, the value -1 is returned. | |
Parameters | |
element | (Selector|Element|jQuery) A string containing a selector, a reference to the element, or a jQuery object whose ordinal value is to be determined. In case a jQuery object is given, the first element of the set is searched. If no argument is given, the index returned is that of the first element of the set within its list of siblings. |
Returns | |
The ordinal value of the specified element within the set or its siblings or -1 if not found. |
To help you understand this method, let’s say that you have the following HTML code:
<ul id="main-menu">
<li id="home-link"><a href="/">Homepage</a></li>
<li id="projects-link"><a href="/projects">Projects</a></li>
<li id="blog-link"><a href="/blog">Blog</a></li>
<li id="about-link"><a href="/about">About</a></li>
</ul>
For some reason you want to know the ordinal index of the list item (<li>) containing the link to the blog, which is the element having the ID of blog-link, within the unordered list having the ID of main-menu.
Note
It’s not a best practice to fill your pages with so many IDs because in large applications it’s hard to manage them and assure that there won’t be duplicates. We used them for the sake of the example.
You can obtain this value with this statement:
var index = $('#main-menu > li').index($('#blog-link'));
Based on what you learned about the parameters accepted by index(), you can also write this statement like this:
var index = $('#main-menu > li').index(document.getElementById('blog-link'));
Remember that the index is zero-based. The first element has index 0, the second has index 1, and so on. Thus, the value you’ll obtain is 2 because the element is the third in the list. This code is available in the file lesson-3/jquery.index.html and also as a JS Bin.
The index() method can also be used to find the index of an element within its parent (that is, among its siblings). This case can be a bit hard to understand, so let’s drill down to see its meaning. The parent of the list item with ID of blog-link is the unordered list main-menu. The siblings are the elements at the same level from the DOM tree point of view (siblings) of blog-link that share the same parent (the unordered list). Given our markup, these elements are all the other list items. The links are excluded because they’re inside main-menu but not at the same level of blog-link. Writing this
var index = $('#blog-link').index();
will set index, once again, to 2.
To understand why calling index() without a parameter is interesting, consider the following markup:
<div id="container">
<p>This is a text</p>
<img src="image.jpg" />
<a href="/">Homepage</a>
<img src="anotherImage.jpg" />
<p>Yet another text</p>
</div>
This time, the markup contains several different elements. Let’s say you want to know the ordinal index of the first img element within its parent (the <div> with ID of container). You can write
var index = $('#container > img').index();
The value of index will be set to 1 because, among the children of container, the first <img> found is the second element (coming after the <p>).
In addition to retrieving the index of an element, jQuery also gives you the ability to obtain subsets of a set, based on the relationship of the items in a jQuery collection to other elements in the DOM. Let’s see how.
Getting sets using relationships
jQuery allows you to get new sets from an existing one, based on the hierarchical relationships of the elements to the other elements within the DOM.
Let’s say you have a paragraph having an ID of description and you want to know the number of its ancestors that are a <div>. With your current knowledge of selectors and methods this isn’t possible. That’s where a function like parents() comes into play. Consider the following code:
var count = $('#description').parents('div').length;
Using parents(), you’re able to retrieve the information you want. This method retrieves the ancestors of each element in the current set of matched elements (which consists of the only paragraph having description as its ID). You can optionally filter the ancestors using a selector, as in the example. Because in your jQuery collection you have just one element (assuming it exists in your page), the result is what you expect.
What if you want to know the number of children of your hypothetical paragraph? This can be easily achieved using selectors:
var count = $('#description > *').length;
But wait! Are you using the same Universal selector we highly discouraged in the previous sections? Unfortunately, yes. A better approach from a performance point of view is to express the same statement using the children() method as follows:
var count = $('#description').children().length;
This method, however, doesn’t return text nodes. How can you deal with this case?
For such situations where you have to work with text nodes, you can employ contents(). The contents() method and children() differ in another detail: the former doesn’t accept any parameters. Returning to our counting example, you can write
var count = $('#description').contents().length;
You know that just counting elements isn’t very useful and we know that you’re impatient to get your hands dirty and start creating awesome effects using jQuery. We ask you to wait a few pages in order to allow us to provide you with a solid knowledge base.
The find() method is probably one of the most used methods. It lets you search through the descendants of the elements (using a depth-first search) in a set and returns a new jQuery object. This new jQuery object contains all the elements that match a passed selector expression. For example, given a set of matched elements in a variable called $set, you can get another jQuery set of all the citations (<cite>) within paragraphs (<p>) that are descendants of elements in the original set:
$set.find('p cite');
Like many other jQuery methods, the find() method’s power comes when it’s used within a jQuery chain of operations. This method becomes handy when you need to constrain a search for descendant elements in the middle of a jQuery method chain, where you can’t employ any other context or constraining mechanism.
Before listing all the methods belonging to this category, we want to show you another example. Imagine you have the following HTML snippet:
<ul>
<li class="awesome">First</li>
<li>Second</li>
<li class="useless">Third</li>
<li class="good">Fourth</li>
<li class="brilliant amazing">Fifth</li>
</ul>
You want to retrieve all the siblings of the list item having class awesome up to but excluding the one having both the classes brilliant and amazing (the fifth). To perform this task, you can use nextUntil(). It accepts a selector as its first argument and retrieves all the following siblings of the elements in the set until it reaches an element matching the given selector. Hence, you can write
var $listItems = $('.awesome').nextUntil('.brilliant.amazing');
What if you want to perform the same task but retrieve only those having the class good? The function accepts an optional second argument, called filter, that allows you to achieve this goal. You can update the previous statement, resulting in the following:
var $listItems = $('.awesome').nextUntil('.brilliant.amazing', '.good');
You can execute this example in your browser by loading the file lesson-3/jquery.nextuntil.html or by accessing the relative JS Bin.
Table 3.1 shows this and the other methods that belong to this category and that allow you to get a new jQuery object from an existing one. Most of these methods accept an optional argument, which will be specified by adopting the usual convention of wrapping it with square brackets.
Table 3.1. Methods for obtaining a new set based on relationships to other HTML DOM elements
Now that we’ve described all these methods, let’s look at a concrete example of some of them.
Consider a situation where a button’s event handler (which we’ll explore in great detail in lesson 6) is triggered with the button element referenced by the this keyword within the handler. Such a situation occurs when you want to execute some JavaScript code (for example, a calculation or an Ajax call) when a button is clicked. Further, let’s say that you want to find the <div> block within which the button is defined. The closest() method makes it a breeze:
$(this).closest('div');
But this would find only the most immediate ancestor <div>; what if the <div> you seek is higher in the ancestor tree? No problem. You can refine the selector you pass to closest() to discriminate which element is selected:
$(this).closest('div.my-container');
Now the first ancestor <div> with the class my-container will be selected.
The remainder of these methods work in a similar fashion. Take, for example, a situation in which you want to find a sibling button with a particular title attribute:
$(this).siblings('button[title="Close"]');
What you’re doing here is retrieving all the siblings that are <button>s and have the title “Close.” If you want to ensure that only the first sibling is retrieved, you can employ the first() method you learned in this lesson:
$(this).siblings('button[title="Close"]').first();
These methods give you a large degree of freedom to select elements from the DOM based on their relationships to the other DOM elements. How would you go about adjusting the set of elements that are in a jQuery collection?
Leave a Reply