For all the same reasons that it’s desirable to segregate style from structure within an HTML document, it’s just as beneficial (if not more so) to separate the behavior from the structure. Ideally, an HTML page should be structured as shown in figure 1.2, with structure, style, and behavior each partitioned nicely in its own niche.
Figure 1.2. With structure, style, and behavior each neatly tucked away within a page, readability and maintainability are maximized.
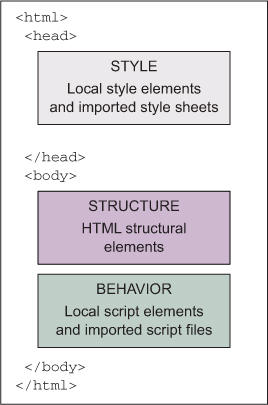
This strategy, known as unobtrusive JavaScript, is now embraced by every major JavaScript library, helping page authors achieve this useful separation on their pages. As the library that popularized this movement, jQuery’s core is well optimized for producing unobtrusive JavaScript easily. Unobtrusive JavaScript considers any JavaScript expressions or statements placed within or among HTML tags in the <body> of HTML pages, either as attributes of HTML elements (such as onclick) or in script blocks placed anywhere other than the very end of the body of the page, to be incorrect.
“But how can I instrument the button without the onclick attribute?” you might ask. Consider the following change to the button element:
<button id="test-button">Click Me</button>
Much simpler! But now, you’ll note, the button doesn’t do anything. You can click it all day long, and no behavior will result. Let’s fix that.
Segregating the script
Rather than embedding the button’s behavior in its markup, you’ll segregate the script by moving it to a script block. Following the current best practices, you should place it at the bottom of the page before the closing body tag (</body>):
<script>
document.getElementById('test-button').addEventListener(
'click',
function() {
document.getElementById('xyz').style.color = 'red';
},
false
);
</script>
Because you’re placing the script at the bottom of the page, you don’t need to use a handler attached to the onload event of the window object, like developers (erroneously) use to do in the past, or wait for the DOMContentLoaded event, which is only available in modern browsers. The DOMContentLoaded event is fired when the HTML document has been completely loaded and parsed, without waiting for stylesheets, images, and so on to finish loading. The load event is fired when an HTML page and its dependent resources have finished loading (we’ll return to this topic in section 1.5.3). By placing the script at the bottom of the page, when the browser parses the statement, the button element exists because its markup has been parsed, so you can safely augment it.
Note
For performance reasons, script elements should always be placed at the bottom of the document body. The first reason is to allow progressive rendering, and the second is to have greater download parallelization. The motivation behind the first is that rendering is blocked for all content below a script element. The reason behind the second is that the browser won’t start any other downloads, even on a different hostname, if a script element is being downloaded.
The previous snippet is another example of code that isn’t 100% compatible with the browsers your project might be targeting. It uses a JavaScript method, addEvent-Listener(), that’s not supported by Internet Explorer 6–8. As you’ll learn later on in this app, jQuery helps you in solving this problem, too.
Unobtrusive JavaScript, though a powerful technique to add to the clear separation of responsibilities within a web application, doesn’t come without a price. You might already have noticed that it took a few more lines of script to accomplish your goal than when you placed it into the button markup. Unobtrusive JavaScript may increase the line count of the script that needs to be written, and it requires some discipline and the application of good coding patterns to the client-side script.
But none of that is bad; anything that persuades you to write your client-side code with the same level of care and respect usually allotted to server-side code is a good thing! But it is extra work—without jQuery, that is.
jQuery is specifically focused on the task of making it easy and delightful for you to code your pages using unobtrusive JavaScript techniques, without paying a hefty price in terms of effort or code bulk. You’ll find that making effective use of jQuery will enable you to accomplish much more on your pages while writing less code. The motto is still “Write less, do more,” isn’t it? Without further ado, let’s start looking at how jQuery makes it so easy for you to add rich functionality to your pages without the expected pain.
Leave a Reply